React
Bar Chart Stacked
Visualize portions of data that are part of a whole.
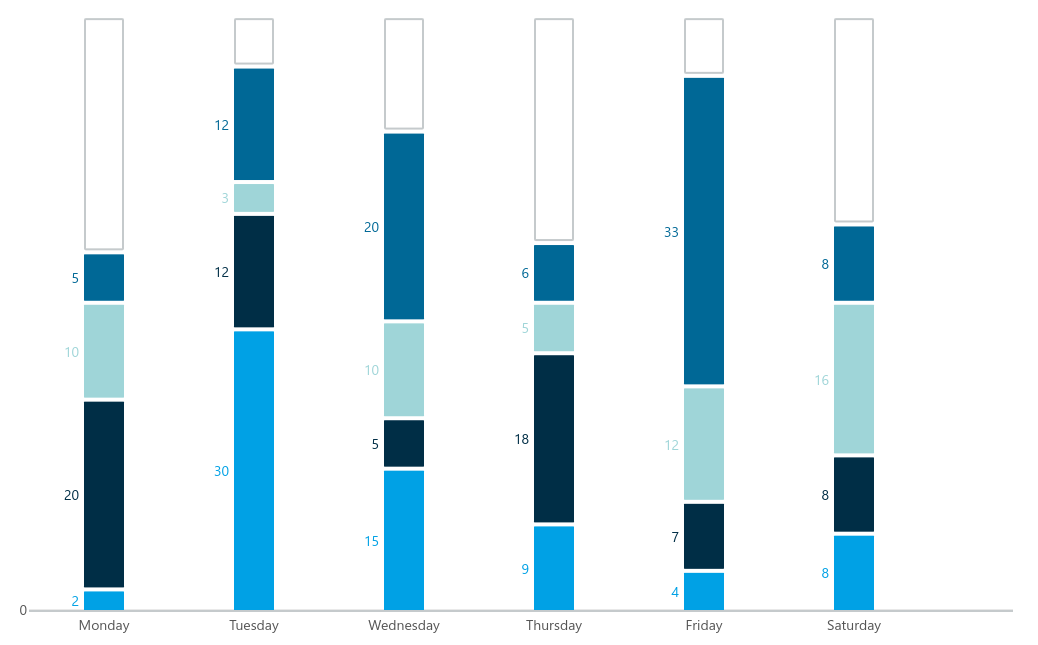
Basic Implementation
To render a stacked bar chart, import the BarChartStacked
component.
The following implementation will generate the chart you can see in the image above:
import { BarChartStacked } from '@meistercharts/meistercharts-react'
export function MyChart() {
const config = {
data: {
categories: [
{ label: 'Monday', values: [2, 20, 10, 5] },
{ label: 'Tuesday', values: [30, 12, 3, 12] },
{ label: 'Wednesday', values: [15, 5, 10, 20] },
{ label: 'Thursday', values: [9, 18, 5, 6] },
{ label: 'Friday', values: [4, 7, 12, 33] },
{ label: 'Saturday', values: [8, 8, 16, 8] }
]
}
}
return <BarChartStacked configuration={config} />
}
For improved performance you should initialize the chart configuration inside a useMemo hook.
Chart Configuration
The data as well as the chart style is configurable via the configuration prop. It accepts an object with the following properties:
Property | Value | Description |
---|---|---|
data | BarChartData | The data to be rendered in the chart. |
style? | BarChartStackedStyle | Style options for the chart. |
On this page